Set Up Advanced Features for the Mobile SDK
This page helps you set up advanced features you can use with the mobile SDK. Examples of advanced features are rich messages (TORM messages), welcome messages, and push notifications. The majority of these features require setup in CXone by an administrator. Then, the developer can implement the features in the chat UI with the SDK. On the developer side, they must initialize the chat, register listeners and delegates, then handle these advanced features.
The developer's main task is implementing these features in the chat UI. They should not be required to add any business logic.
Attachments
Both contacts The person interacting with an agent, IVR, or bot in your contact center. and agents can send messages with attachments, like images, videos, or PDFs. The files display as a preview in the conversation with the other messages. After the message sends, the recipient can:
-
Tap the file to view it with their full screen.
-
Tap videos to play with their full screen.
-
Tap audio files to play the audio.
In your chat channel's settings in CXone, you can define file size and type restrictions. When a channel is created, it has a default set of limitations. You can change the defaults to meet your channel's needs. Specifying file types requires that you know the MIME A 2-part identifier that specifies the general category and subtype for a file. For example, application/msword. More secure than relying on file extension to determine file type. type for the files you want to allow or restrict. If the contact tries to upload a file type that you don't support, they receive an error message indicating that it's unsupported.
iOS has a maximum file size limitation of 40 MB. Android has a maximum 12 MB limitation for contacts.
In the SDK, you can handle attachments starting with the ChannelConfiguration object.
Pre-Chat Surveys
Displays a form to obtain essential information from the contact before they start a chat. These are commonly used to gather basic contact information. The form has four types of elements that can be required or optional for the contact:
-
Plain text field
-
Email address
-
Drop-down selector
-
Hierarchical selection
You create the form in CXone and assign it to the chat channel of your app. The form uses custom fields that you also set up in CXone. You can find references to contact and case custom fields in the SDK. These custom fields can also display in the agent application. You must use the SDK to implement the form functionality.
The SDK does not perform any validation, this must be done on the backend by CXone. For example, if the contact enters an invalid email, the SDK propagates the error to the SDK integration application. Information from the form then displays to the agent in their agent application.
Welcome Messages
This is an auto-generated message that contacts see when they start a chat. It typically gives the contact a prompt like describing their issue or choosing a set of options. These are set up in CXone as an engagement action and rule.
You can personalize the welcome message with custom fields. For example, if you display a pre-chat survey to gather personal details, you could pull the contact's name into the welcome message. In the Main body text of the action, you would add the custom field as a variable. You can include conditions in these variables to display a unique message, like {{ customer.location == Athens }} Did you visit the Parthenon?. If the condition is not met, the {{ fallbackMessage | <enter message here> }} variable lets you add an alternative message to display.
Rich Messages
These are messages that offer more than simple text. They are interactive elements like lists, rich links, and so on. They help engage contacts and make the chat experience more interesting. These are truly omnichannel rich messaging (TORM) messages. TORM messages offer the following:
- Rich Link: A simple URL link enriched with an image. The image displays like an image attachment with the URL at the bottom. The URL can be a web link that opens the contact's browser, or a deeplink
A URL that opens a specific area or page within a mobile app. For example, an agent could send a deeplink to the contact that directs to a page in the app that shows an account balance. that opens a specific page within your app.
- Quick Reply: Displays a list of canned responses that the contact can choose from. This is similar to a menu or list picker, but when the contact selects an option, the chat sends a template message. For example, you could display this message with three options: Sales, Support, and Account. If the contact selects Support, the chat can send a canned response about your support options, like Tap here to chat with a support agent or Call us at 1-800-867-5309. These replies speed up the process to help contacts with common requests. Contacts can only interact with a quick reply once.
- List Picker: Displays a list of options with introductory text to explain the list. Contacts can interact with the list more than once; they can select different list items.
You can set up TORM messages in CXone. One benefit of these messages is that you can set them up in one place and use them across all digital channels. If your organization already has other digital channels set up, you may already have existing messages that you can access with the SDK.
Push Notifications
These are notifications that display on the contact's phone when they're not using your app. These notifications inform contacts when an agent sends a chat message when they aren't using your app. The notification populates in the phone's list of notifications, like missed calls or calendar events. The contact can tap the notification to open the chat. You can customize which view or screen opens when the chat opens. For example, you could fetch the thread list to display conversations, or open a specific message thread.
Setting up push notifications requires a Firebase API key, platform-specific certificates, configuration in CXone, and implementation with the SDK.
-
For Android, set up Firebase messaging to receive a device token from the mobile device. See the Firebase documentation
for instructions.
-
For iOS, create a P12 certificate file for push notifications. You can create these in the certificates section of developer.apple.com
. You can also see the Amplify dev center
for more information.
-
Add the API key and certificate to your chat channel:
- In CXone, click the app selector
and select ACD.
- Go to DFO > Points of Contact Digital > Chat > locate your chat channel > Push Notifications.
- Enable Push notifications are enabled.
- Enter a Title, which appears as the main text on the push notification.
- Enter Body text, which is the secondary text that explains the notification. For example, you could enter New message from CXone!.
- Enter a Deeplink URI Link if you want the contact to be directed to a specific page in your app when they click the notification.
- For Android notifications, enable Enabled for Android and enter your Android API key.
- For iOS notifications, enable Enabled for iOS and upload your P12 certificate.
- In CXone, click the app selector
- Configure the engagement rule and action in CXone. These determine the conditions for when CXone sends the push notification to the contact.
- Implement the notification in your SDK.
The README files in the iOS and Android repositories provide additional information for setting up these notifications.
Proactive Actions
Proactive actions are notifications that you can display when the chat is initialized and theWebSocket is connected. Currently, you can display an inactivity message to the contact. This occurs when the WebSocket sends an inactivity event when the chat has been idle for a certain amount of time. When this event fires, you can display a message prompting the contact to engage with the chat. Both iOS and Android SDKs have proactive action functions that interact with a proactive action. These let you display it or let the contact tap on the notification to prompt some behavior.
OAuth 2.0
You can require your app users to sign in with an existing account before they start a chat. The SDK lets you use any authentication provider that uses OAuth2.0, like Okta. Requiring authentication of your contacts provides the following benefits:
-
You can automatically pull in their contact information from their credentials, like first name. This requires field mapping between yourauth provider's response and fields in the CXone chat channel.
-
Contacts can see an archive of previous conversations.
-
Contacts can log in with different devices. Conversations are tied to their account, which lets them access their archived conversations on multiple devices.
Setting up OAuth requires configuration in CXone. You must enter certain URLs or URIs in the chat channel. CXone uses these to obtain an access token and user details from yourauth provider. This configuration is also where you map fields to pull contact information into the chat. The getting started documentation in the SDK repository provides instructions for setting up OAuth in your app.
Currently, the SDK supports OAuth2.0 through Amazon or other providers with a similar OAuth implementation.

The following image shows an overview of the different authentication steps. An explanation of the steps appears below the diagram. You are responsible for the first few steps in the light green color. CXone handles the latter steps, indicated in dark blue.
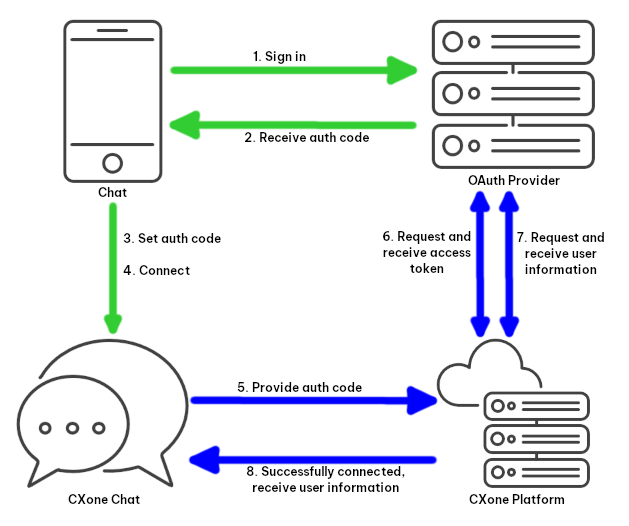
-
Prompt the contact to sign in with your authentication provider.
-
The provider responds with anauth code. This must be anauth code, which is later exchanged for anauth token or access token.
-
Set the auth code with the mobile SDK so it can eventually pass it to CXone.
-
Connect to the CXone chat using the SDK. This can happen anytime after setting the auth code; users may sign in when opening your app, and may open the chat later.
-
The SDK provides the auth code to CXone.
-
CXone uses the auth code to request and receive the access token from your authentication provider.
-
CXone requests and receives the user's information from your authentication provider.
-
The connection is successful and user information received.
Visitor Events
CXone can collect event data on what contacts do in the chat. These events can also be used to trigger certain functionality, similar to other features. You can also use your own reporting tool to utilize this data.