Customize Chat Channels Using JavaScript
You can customize live chat Agents and contacts interact on a real-time basis and chat messaging
Asynchronous chat in which contacts send a chat message anytime and wait for a reply with JavaScript. CXone provides default customizations and their correlating JavaScript API calls. You must include these API calls with the script tag that adds the chat to your website. To add these features, you need access to your website's code. This page explains where to add the JavaScript and the exact lines of code to include. You should also have familiarity with JavaScript or web development to confidently use the information on this page.

The Jungle, a subsidiary of Classics, Inc., runs a gift store as part of its website. The administrator, Mowgli Kipling, has set up the following API calls to help his chat agents work with gift store customers:
- Custom visitor variables collect information about the colors of items the contact views while on the gift store pages. This information is then available to the agent when the contact initiates a chat.
- Custom visitor events are recorded for each product a contact clicks on. After five product clicks and no clicks of the Buy button, a proactive "Having Trouble Deciding?" pop-up offers the contact a chat.
You can customize the following aspects of your chat channels A way for contacts to interact with agents or bots. A channel can be voice, email, chat, social media, and so on.:
- Chat behavior: Customize how the chat window behaves. This includes opening and closing the chat window or enabling game mode. Game mode lets customers play the game Snake while waiting for an agent.
- Chat information: Customize the information displayed in the chat window. This includes things like the customer's name, agent image, or activity status.
- Custom fields, labels, and phrases: Define custom fields or customize field labels. You can also configure alternate text for the automated messages shown to contacts.
- Chat window appearance: Customize the appearance of the chat window. You can change things like the size and position of the window on the webpage.
- Custom CSS: Define custom CSS for the chat window.
- Proactive actions: Customize the experience with proactive actions based on customer behavior.
- Other functions: Other things you can configure include:
- Having the host environment listen for certain events.
- Integrating digital chat channels into iOS or Android apps.
- Enabling OAuth authentication.
Currently, all JavaScript APIs support a Brand Embassy loader for each call. If you use standalone CXone chat on your website, all of these calls also support a new CXone loader. Eventually all JavaScript APIs for chat will use the CXone loader instead of Brand Embassy.
The structure of the JavaScript call is different depending on which loader you use. You cannot use both structures at the same time. Only use one or the other depending on which loader you use.
If you use CXone Guide to implement chat on your website, use the CXone loader. Not all of the calls on this page are supported in Guide, but the CXone Guide documentation lists all supported JavaScript calls.
If you use the CXone loader, either through digital chat or Guide, structure calls like this:
cxone('chat','enableChatAlwaysOnline');
If you use the Brand Embassy loader, structure calls like this:
brandembassy('enableChatAlwaysOnline');
Add JavaScript Calls to a Chat Channel
If you haven't yet done so, set up a live chat or chat messaging channel. Test the channel to ensure that it works with the default settings before you add customizations. Set up chat customizations in a test environment before releasing them into your production environment. This will help minimize disruptions to your website visitors. It will also help ensure that your customizations work as intended.
Copy the script and API calls exactly as shown. Any unexpected changes could cause your channel to malfunction.
- Click the app selector
and select ACD.
-
Go to Digital > Points of Contact Digital.
- Under Your Channels, click Website Script.
- Copy the JavaScript code from the page and paste it into a text editing program like Notepad++. Be sure to include the opening and closing <script> tags.
-
In Notepad++, just above the closing </script> tag of the code you pasted in, add the JavaScript calls you want to use. In the following example image, the comment indicates where to add calls. The supported calls you can use are described in the rest of this page.
-
Copy and paste the entire script, including the opening and closing <script> tags, into the header of a page of your website.
-
Visit the modified page and send test chat messages to make sure your customizations work as intended.
-
When it is fully tested and works as expected, copy the final script into the header of each page that should have the chat widget.
Chat Behavior JavaScript Calls
The calls in this section allow you to change how chat windows behave.
Open Chat Window
Opens a chat window automatically. The default behavior is for chat windows to open when a contact clicks on the chat icon.
brandembassy('openChatWindow');
Close Chat Window
Closes a chat window automatically. The default behavior is for chat windows to close when a contact clicks on the chat icon.
brandembassy('hideChatWindow');
Chat Always Online
Displays the chat window as if there are agents available, even when no agents are online.
brandembassy('chat','enableChatAlwaysOnline');
Persist Custom Fields from Previous Chat
You can choose if you want custom fields to persist or be purged from local storage after the interaction has been initiated. This lets you select which custom fields continue to appear across all interactions with a contact. The 'field_id' refers to the value of the Ident field when creating a custom field.
cxone('chat','enablePrecontactSurveyFieldAutoFill'('field id');
Send Postback Data from Adaptive Card
Studio actions and bots can send Adaptive Cards to contacts via chat. In order for digital chat to send postback data to Studio or a bot, you must enable a handler. An Adaptive Card handler functions within the bot or Studio action to manage and respond to engagements with Adaptive Cards. The handler activates when a user interacts with components of an Adaptive Card, such as clicking a button or selecting an option from a drop-down menu. The handler deciphers the user's input and determines the appropriate response or action to take. Add the default handler command and the send message command, so that Studio or bots can respond accordingly or store the Adaptive Card data for reporting purposes. You can also override the default handler with a custom handler command.

<script>
(function (i, s, o, r, g, v, a, m) {
g = v ? g + '?v=' + v : g; i['CXoneDfo'] = r;
i[r] = i[r] || function () {(i[r].q = i[r].q || []).push(arguments)}; i[r].l = +new Date(); i[r].u = g;
a = s.createElement(o); m = s.getElementsByTagName(o)[0]; a.async = true;
a.src = g + '?' + Math.round(Date.now() / 1000 / 3600); m.parentNode.insertBefore(a, m);
})(window, document, 'script', 'cxone', 'https://web-modules-de-na1.staging.niceincontact.com/loader/1/loader.js');
cxone('init', '1032');
cxone('chat', 'init', 1032, 'chat_5184dc2e-0c75-4265-8a2b-4221c5aebfe1');
cxone('chat', ‘adaptiveCardOnExecuteAction’, 'button1Submit', (action, chatSdkInstance) => {
let messageContent = {
type: "TEXT",
payload: {
text: action.title
},
postback: action.id
}
chatSdkInstance.sendMessage(messageContent)
});
</script>
Add default Adaptive Card handler command:
adaptiveCardOnExecuteAction(actionId: string, callback: (action: node_modules/adaptivecards/src/card-elements.ts::Action) => void)
Send message command:
sendMessage(messageContent: MessageContent)
Create custom handler command:
{
"acType": "Action.Execute", // type of action Action.Execute or Action.Submit
"acVerb": "null|string", // only if `verb` is provided in the Action
"acActionId": "null|string" // only if id is provided in the Action
"acData": { // `data` are provided in Action
"firstName": "John",
"lastName": "Doe",
"_messageText": "Submitted something for you"
}
}

{
...,
"messageContent": {
"type": "TEXT",
"payload": {
"text": "Submitted something for you", // use Action.Title if _messageText is not provided (in case Action.Title is not provided, send Action.type)
},
"postback": "{\"acType\":\"Action.Execute\",\"acData\":{\"firstName\":\"John\",\"lastName\":\"Doe\",\"_messageText\":\"Submitted something for you\"}}"
}
}
}

{
"thread": {
"idOnExternalPlatform": "788a67af-263d-48b1-b67e-eb75838c6509"
},
"messageContent": {
"type": "ADAPTIVE_CARD",
"payload": {
"$schema": "http://adaptivecards.io/schemas/adaptive-card.json",
"type": "AdaptiveCard",
"version": "1.4",
"body": [
{
"type": "TextBlock",
"text": "Present a form and submit it back to the originator"
},
{
"type": "Input.Text",
"id": "firstName",
"label": "What is your first name?"
},
{
"type": "Input.Text",
"id": "lastName",
"label": "What is your last name?"
}
],
"actions": [
{
"type": "Action.Execute",
"title": "Fire!",
"data": {
"_messageText": "Submitted something for you"
}
}
]
}
}
}
Set Authorization Code
Sets an authorization code for an OAuth flow. OAuth is an authentication protocol. It allows you to let one application interact with another on your behalf without giving away your passwords. This must be called before chat initialization.
brandembassy('setAuthorizationCode', 'authorization_code');
Auto-Start a New Chat Session
Creates a new chat messaging Asynchronous chat in which contacts send a chat message anytime and wait for a reply or live chat
Agents and contacts interact on a real-time basis session if one is not currently in progress. Use this call on lower-traffic pages of your website to avoid overwhelming your agents with contacts.
Digital Experience creates a new case and adds it to the queue when the first message of the chat session is sent. A hidden, automated message is sent on the customer's behalf when the chat session starts. This decreases the amount of time contacts wait for an agent by starting the process sooner. Chat sessions start when a contact clicks the chat icon to begin a conversation or when the chat window opens for auto-started chat sessions. Although the initial message is hidden from the customer, the agent can see it.
You can customize the text of the initial, hidden message.Auto-starting a chat session skips the precontact survey form. Because of this, you need to use other methods to collect information about contacts. For example, you can have agents enter the information manually into the customer card. You can also configure customer authentication using an OAuth integration.
Routing for auto-started cases occurs according to the routing queues The system uses routing queues to determine which agents to route cases to. Your system administrator creates routing queues so that certain cases are routed to agents with expertise in that type of case. configured in Digital Experience. For live chat sessions, if no agents are available, the chat window displays a Waiting for available agent message.
To auto-start a chat session, use these calls in the order shown:
brandembassy('openChatWindow');
brandembassy('autoStartSession');
If you add additional calls to the script, autoStartSession must always be the last call in the script. For example:
// set customer name (as this is required field)
brandembassy('setCustomerName', 'Elizabeth');
// open chat window so user can start to chat
brandembassy('openChatWindow');
// and finally start the new chat session (without the pre-chat form)
brandembassy('autoStartSession');
Enable Concurrent Chat Sessions
You can let contacts start multiple chat sessions in the same browser. If they're in an active chat in one browser tab, this lets the contact begin a second chat in a new browser tab or window.
To enable this behavior, you must specify how the chat data is stored. You have two options:
-
localStorage: Data persists in the browser after the tab or window is closed. This is the default storage method. If multiple chat sessions are simultaneously open in different tabs, they are all considered the same interaction. The chat conversation and experience is the same across all tabs.
-
sessionStorage: Data is stored for the individual tab or window, then cleared when the tab or window closes. The user can create multiple chat sessions across different tabs or windows. The chat session in each tab is considered a unique interaction.
To enable concurrent chat sessions, add the storageType key with a value of sessionStorage, as shown in the following example.
If you use the localStorage option and still want to purge the conversation history, you can create an event listener that removes the customerID after the chat ends.
brandembassy( 'init', 'MY_BRAND_ID_123', 'MY_CHANNEL_ID_321', undefined, { storageType: 'sessionStorage', }, );
Purge Conversation History
Removes conversation history after a chat conversation ends. This may be useful if you use the default storage option of the chat, local storage. This storage option stores data even after the browser instances close. In this case, you can specifically remove the conversation history by clearing the customerID from the local storage. The following example creates an event listener to listen for the caseStatusChanged event from the chat. This indicates that a chat session ended. Afterward, the removeItem command is called, which removes the customerID from the browser's local storage.
brandembassy('onPushUpdate', ['CaseStatusChanged'], pushUpdatePayload => { if (typeof pushUpdatePayload === 'undefined' || typeof pushUpdatePayload.data === 'undefined') { return; } const { status } = pushUpdatePayload.data.case || {}; if (typeof status === 'undefined') { return; } // When a previous case has been closed, this will set the custom fields again. if (status === 'closed') { localStorage.removeItem('_BECustomerID') ); } });
Customize the Initial Automated Contact Message
Digital Experience creates a new case and adds it to the queue when the first message of the chat session is sent. A hidden, automated message is sent on the customer's behalf when the chat session starts. This decreases the amount of time contacts wait for an agent by starting the process sooner. Chat sessions start when a contact clicks the chat icon to begin a conversation or when the chat window opens for auto-started chat sessions. Although the initial message is hidden from the customer, the agent can see it.
The default message is the text on the button that the customer clicks to start the conversation. You can customize the text of the initial, automated message in three ways:
- Override the default button text.
brandembassy('sendFirstMessageAutomatically', 'Hello');
brandembassy('setFirstAutomatedMessageContent', 'Hello, I have a question');
- Change the message text on the Translations page.
Make the Initial Automated Message Visible to Contacts
If you want the contact to be able to see the initial automated message, add this call to your script:
brandembassy('hideFirstSentMessage', false);
Delay Case Creation
Digital Experience creates a new case and adds it to the queue when the first message of the chat session is sent. A hidden, automated message is sent on the customer's behalf when the chat session starts. This decreases the amount of time contacts wait for an agent by starting the process sooner. Chat sessions start when a contact clicks the chat icon to begin a conversation or when the chat window opens for auto-started chat sessions. Although the initial message is hidden from the customer, the agent can see it.
You can configure Digital Experience to wait for the contact's actual first message before creating a case using this call:
brandembassy('sendFirstMessageAutomatically', false);
Enable Game Mode
Allows contacts to play the game Snake while waiting for an agent.
brandembassy('allowGameMode');
Automated Satisfaction Survey
A satisfaction survey can be automatically detected and displayed in a pop-up. This enables or disables them to be detected and displayed or not.
brandembassy('automatedSatisfactionSurveyModal', 'true');
brandembassy('automatedSatisfactionSurveyModal', 'false');
//if not defined, the default is 'true'
Chat Information JavaScript Calls
These calls allow you to customize the information that's available to contacts during a chat session.
Get Customer Identity ID
Returns the unique identifier of the customer in the chat.
brandembassy('getCustomerIdentityId');
Hide Precontact Survey Form
The precontact survey form appears at the start of a chat messaging Asynchronous chat in which contacts send a chat message anytime and wait for a reply session. It collects information about the contact, such as their name. If you want, you can hide the precontact survey form and have the chat window appear as soon as a contact starts a chat messaging session.
brandembassy('hidePreSurvey');
Hide Queue Counter
The queue counter lets a contact know how soon an agent will be able to help them based on where they are in the agent's queue. You can hide the queue counter after the contact has been successfully routed to an agent. This allows agents to remove customer cards from their inbox without contacts noticing.
brandembassy('hideQueueCounterAfterAssignment');
brandembassy('hideAssignedAgent');
brandembassy('hideSystemMessages');
Mute Chat Notification Audio
Allows you to turn the chat notification sound off. The chat notification sound is on by default. This needs to be configured regardless of other chat sound settings. You can change the value to 'false', to turn the chat notification audio back on.
brandembassy('muteAudioNotification', 'true');
// OR
brandembassy('muteAudioNotification');
Override Browser Language Translations
Overrides the user's browser language settings or a customized locale to a set translation. For example, you can ensure that certain text always appears in English regardless of a contact's browser language settings.
brandembassy('init', 'brandId', 123, 'locale');
Set Activity Status
Changes the message in the activity bar. For example, you could use it to communicate your response time for a chat messaging session. This could be helpful because chat messaging is an asynchronous form of chat, so contacts could have to wait to hear from an agent.
brandembassy('setStatusMessage', 'We typically reply within 3 hours.');
Set Agent's Image
Dynamically changes the agent image displayed in the chat window. By default, Digital Experience uses the image in the agent's profile. When you include this call in your script, it uses the image specified by the URL in the code snippet. Replace the example URL with the URL of the image you want the chat to use.
brandembassy('setAgentImage', 'http://classics.com/images/agents/elizabeth_bennet.png');
Set Customer ID
Sets a unique identifier for the customer in the chat.
brandembassy('setCustomerId', 'customer_id');
Set Customer Name
Customer Name is a field on the precontact survey form, which contacts are required to fill in prior to chatting with an agent on a chat messaging Asynchronous chat in which contacts send a chat message anytime and wait for a reply channel. This call dynamically fills the field.
brandembassy('setCustomerName', 'Elizabeth');
Set Date Format
Can be set to use absolute date format (12/03/2035) or relative date format (today, Monday).
brandembassy('setDateFormat', 'date_format');
// date_format can be 'absolute' or 'relative'
Set Locale
Sets the locale for translations in the chat window.
brandembassy('setLocale', 'locale');
Show Case Information
Only for chat messaging Asynchronous chat in which contacts send a chat message anytime and wait for a reply channels. Shows case information to the contact.
brandembassy('showCaseInfo');
Show/Hide the Send Transcript Button
If you make the Send Transcript button visible, you must configure the chat channel to allow contacts to send the chat transcript to themselves. Configure this option for chat messaging or live chat.
brandembassy('hideSendTranscript');
brandembassy('showSendTranscript');
Show/Hide System Messages
Shows or hides system messages like agent assignment history.
brandembassy('chat','hideSystemMessages');
brandembassy('showSystemMessages');
Terms of Use
You can make a terms of use document available to contacts from within the chat window. This function adds a button to the window. You can customize the button's label and style, and what happens when contacts click it. The on-click action can show the terms of use as:
- An internal window
- An external link
// Internal Modal Window
brandembassy('showTermsOfUse', 'Show terms of Use', 'modal' , 'Our terms of use are ...');
// External Link
brandembassy('showTermsOfUse', 'Show terms of Use', 'externalLink' , 'http://yourdomain.com/terms-of-use');
// If you want to explicitely disable this feature, you can use
brandembassy('hideTermsOfUse');
To customize the appearance of the button or the internal window, use the CSS API calls described on this page.
Custom Fields, Labels, and Phrases
Create and Populate Custom Fields
The custom fields you can use with this call are the same as the ones you can use in Customer card. If you use these calls before opening a chat window, the fields in the precontact form are populated with the data you include in the JavaScript call.
To define the value of a case custom field:
brandembassy('setCaseCustomField', 'ident_of_custom_field', 'value_of_custom_field');
To define the value of a customer card custom field:
brandembassy('setCustomerCustomField', 'ident_of_custom_field', 'value_of_custom_field');
Customize Chat Labels and Phrases
You can customize many of the labels and phrases that digital chat channels use, as well as define the customizations. If you need to dynamically change the phrases or screen labels, use these JavaScript calls.
To change a single message or label:
brandembassy('setTranslation', 'key', 'your translation');
To change multiple messages or labels:
brandembassy('setTranslations', {
key1: 'your custom label',
key2: 'your custom message'
});

Curly braces indicate variables that the system replaces with actual data. For example, {caseNumber} is replaced with the case number of the contact's chat session.
Key | Default label/Message |
---|---|
allAgentsForQueueAreBusy | All our agents dedicated to your queue are currently busy. There {queue, plural, one {is} other {are}} <strong>{queue, number}</strong> {queue, plural, one {person} other {people}} ahead of you in the queue. |
beginButton |
Begin chat! |
cancel | Cancel |
caseNumber | Case #{caseNumber} |
change | Change |
chattedWith | You just had a chat with |
commonErrorText | There was an unexpected error. Please try again later. |
done | Done |
dragAndDropDropzone | Drag & drop files here, to send them |
dragAndDropDropzoneRejected | Impossible to upload file :( |
EmailLabel | |
endChat | End chat |
endChatTitle | Are you sure you want to end this conversation? |
endGame | End game |
ending | Ending chat... |
fileSendingFailed | File sending failed |
getTranscriptDescription | Send transcript of this conversation to the following email address: |
getTranscriptLink | Get chat transcript |
invalidToken | Invalid token |
ipAddressBlocked | IP address is blocked |
loading | Loading ... |
loadPreviousButton | Load previous conversations |
messageLabel | Message |
networkErrorText | There was a network error. Please try again. |
newCase | New case |
noAgentOnlineForQueue | No agent is available for your queue at the moment. |
offline | Offline |
offlineFormDesc | We are not available at the moment |
offlineFormSuccessMsgHead | Thank you! |
offlineFormSuccessMsgSub | Your email was successfully sent. We'll get back to you soon. |
online | Online |
onlineFormText | To start a chat, please tell us your name. One of our agents will start helping you immediately. |
pleaseSelect | Please select... |
poweredBy | Powered by |
preparingSession | Preparing session... |
replyBoxPlaceholder | Write here, press<enter> to send |
retry | Retry |
sendFileTextSize | Please upload files smaller than {filesize} |
sendFileTextSupportedFormat | supported formats. |
sendFileTextSupportedFormatDesc | Images, Videos, {fileFormats} |
sendMessageButton | Send message |
sendNewEmail | Send new email |
sendTranscript | Send transcript |
snakeWaiting | You're {queue} in queue. |
startChatInPopup | Ask us! |
startNewChat | Start a new chat |
statusReconnecting | Trying to connect… |
surveySuccesfullySent | Thanks for your feedback! |
systemChattingWith | You are now chatting with {name} |
topic | Topic |
transciptSentFailed | Send failed. |
transciptSuccesfullySent | Transcript successfully sent! |
tryAgainButton | Try again |
unexpectedError | Unexpected error. |
validationInvalidEmail | E-mail address is not valid |
validationMandatory | This field is mandatory |
validationShorterName | Please use shorter name |
validationShortMessage | Your message is too short |
waiting | Waiting... |
waitingDescription | One of our agents will talk to you shortly. |
waitingFooter | Hit <spacebar> to shorten the waiting. |
waitingForAgent | Waiting for agent... |
waitingInQueue | Waiting for agent. You're {queue} in queue. |
yourNameLabel | Your name |
Translate Custom Fields
You can translate custom fields displayed in the chat window.
brandembassy('chat', 'setCustomTranslations', {
myCustomFieldATranslationKey: "Custom field value A",
myCustomFieldBTranslationKey: "Custom field value B",
});
Chat Window Appearance Calls
You can modify the appearance of the chat window.
Close Chat Icon
When set to true, an X icon is displayed on the window instead of the End Chat option in the settings menu.
brandembassy('enableCloseIcon', true);
Hide Assigned Agent
brandembassy('hideAssignedAgent');
Hide Header
brandembassy('hideHeader');
Hide Pop-Ups
brandembassy('hidePopups');
Show Customer Avatar
brandembassy('showCustomerAvatar');
Show the Send Button
brandembassy('chat','showSendButton');
Size
Customizes the width and height of the chat window. You can use absolute or relative units supported by CSS specification.
brandembassy('setWindowWidth', '100%');
brandembassy('setWindowHeight', '100%');
Full Display Mode
brandembassy('setFullDisplay');
// OR
brandembassy('chat','setWindowWidth', '100%');
brandembassy('chat','setWindowHeight', '100%');
Position and Offset
The setPositionX call defines the left or right side of the screen as the starting position. The setOffsetX and setOffsetY calls define how many pixels the chat window is offset horizontally and vertically from the starting point, respectively.
// set horizontal starting position
brandembassy('setPositionX', 'right');
// set horizontal and vertical offset
brandembassy('setOffsetX', '40'); // default = 20
brandembassy('setOffsetY', '40'); // default = 20
Set Character Limit for Reply Box
brandembassy('setReplyBoxLimit', '280'); // default = null
Custom CSS with Data Selectors
You can customize the chat window appearance by adding your own CSS. Specify the part of the chat window you want to style with a data-selector attribute. The drop-down below contains a list of all the components that you can select with the data-selector attribute. Data selectors allow you to specify the part of the chat window you want to apply the custom CSS to. After the data-selector, add your styling inside curly braces. If you want to style multiple elements, you must add all your CSS in a single JavaScript call. If you use the same JavaScript call multiple times, the last JavaScript call will override all previous CSS. You can also customize CSS for chat directly in CXone.
To add custom CSS with data selectors, use this JavaScript call as a reference:
brandembassy('setCustomCss', '[data-selector="CUSTOMER_MESSAGE_BUBBLE"] {color: white !important; background: black !important}
[data-selector="CONTENT"] {background: green !important}`);

- ACTIVITY_BAR
- AGENT_MESSAGE
- AGENT_MESSAGE_BUBBLE
- AVATAR
- CONTENT
- CUSTOMER_MESSAGE
- CUSTOMER_MESSAGE_BUBBLE
- DELIMITER
- DROPDOWN_MENU
- HEADER
- INPUT
- IS_TYPING
- MESSENGER
- POPUP
- PRECONTACT_SURVEY
- PRECONTACT_SURVEY_DESCRIPTION
- PRIMARY_BUTTON
- REPLY_BOX
- SECONDARY_BUTTON
- SEND_BUTTON
- TEXTAREA
- TEXT_BUTTON
- WIDGET
- WINDOW
Apply custom CSS components using the data-selector attribute only. Don't apply it to class selectors or other parts of the JavaScript code, such as in this example:
.Widget__Widget___1qQCf { background: red; }

brandembassy('setCustomCss', '[data-selector="CUSTOMER_MESSAGE_BUBBLE"] {color: white !important; background: black !important};');
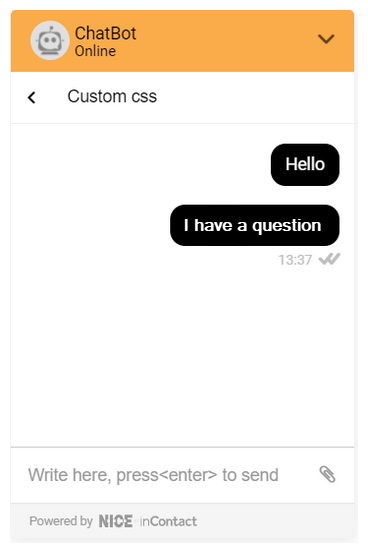
Window components can consist of multiple nested blocks. The component selectors listed in this section name the root of each component. If you want to apply CSS to one of the nested blocks of a component, use the developer's console to inspect the component so you can select the correct block.
Proactive Actions
The calls in this section allow you to proactively engage customers in a chat based on their behavior.
Execute Trigger
Starts the Workflow Automation trigger defined by the trigger_id.
brandembassy('executeTrigger', 'trigger_id');
Set Visitor Event
Stores the defined customer behavior (the event) for reporting purposes. When this call is used with the CXone Mobile SDK, event_type is changed to custom_event_type.
brandembassy('setVisitorEvent', 'event_type', 'custom_event_data');
Set Visitor Variable
Stores the defined customer information for reporting purposes.
brandembassy('setVisitorVariable', 'variable_identifier', 'variable_value');
Store Conversion
Stores the customer behavior as a conversion for reporting purposes.
brandembassy('storeConversion', 'conversion_type', 'conversion_value', 'conversion_date');
// conversion_date is optional, default is now
Web Visitor Tags
Content in this section is for a product or feature in controlled release (CR). If you are not part of the CR group and would like more information, contact your CXone Account Representative.
You can tag contacts that exhibit certain behaviors on your website. This feature uses tags from Digital Experience and requires Guide.
To add a tag to a contact:
brandembassy('assignTag', 'tagID');
To remove a tag from a contact:
brandembassy('removeTag', 'tagID');
Integrate with Third-Party Applications
The calls in this section allow you to receive push notifications when certain chat events occur.
Recognize Active Threads in the Chat Window
brandembassy('getOngoingThreads');
Recognize Ongoing Contact in the Chat Window
brandembassy('getOngoingContact')
Receive Push Notification for Specific Chat Events

Use any of the following inputs to replace 'event' in the examples below.
-
CaseToRoutingQueueAssignmentChanged
-
PageViewCreated
-
MessageCreated
-
MessageDeliveredToUser
-
MessageDeliveredToEndUser
-
MessageSeenByUser
-
MessageSeenByEndUser
-
MessageReadChanged
-
MessageAddedIntoCase
-
CaseInboxAssigneeChanged
-
CaseCreated
-
CaseStatusChanged
-
SenderTypingStarted
-
SenderTypingEnded
-
MessageNoteCreated
-
ContactRecipientsChanged
To receive a notification for one chat event:
brandembassy('onPushUpdate', 'event', callback);
To receive a notification for more than one chat event:
brandembassy('onPushUpdate', ['event', 'event', 'event'], callback);
To receive a notification for all chat events:
brandembassy('onAnyPushUpdate', callback);
Integrate to Android or iOS Applications
You can integrate digital chat into Android or iOS apps. The native application must use WebView where the JavaScript initialization code is added. This example code uses the JavaScript from this page to modify the look and behavior of the chat function:
<script async type=”text/javascript”>
(function(i,s,o,r,g,v,a,m){g=v?g+'?v='+v:g;i['BrandEmbassy']=r;
i[r]=i[r]||function(){(i[r].q=i[r].q||[]).push(arguments)};i[r].l=+new Date();
a=s.createElement(o);m=s.getElementsByTagName(o)[0];a.async=1;
a.src=g+'?'+Math.round(Date.now()/1000/3600);m.parentNode.insertBefore(a,m)
})(window,document,'script','brandembassy','https://livechat-static.brandembassy.com/3/chat.js');
//init of Livechat
brandembassy(‘init’, BRAND_HASH);
// hiding unwanted UI elements
brandembassy('hideHeader');
brandembassy('hidePopups');
// stretching livechat to full width and height
brandembassy('setFullDisplay');
// Customer's name
brandembassy('setCustomerName', 'CUSTOMER_NAME');
// Other optional Custom Fields
brandembassy('setCustomField', 'CUSTOM_FIELD_IDENT', 'CUSTOM_FIELD_VALUE');
// Start Chat
brandembassy('openChatWindow');
brandembassy('autoStartSession');
</script>